THE BASIC C LANGUAGE
object oriented programming.
Encapsulation.
Polymorphism.
CPP Preprocessor Directives.
Comments.
CPP Data types
New and Delete expressions
Type convertions.
Object oriented programming: It is nothing but doing the programs with the help of objects. So first of all we have to know what is an object? How it is implemented in C++ programming? All these details are given below.
CLASS: A class is an expanded concept of a data structure: instead of holding only data, it can hold both data and functions.
OBJECT: An object is an instantiation of a class. In terms of variables, a class would be the type, and an object would be the variable.
By using these objects we can access the class members and member functions.
Classes are generally declared using the keyword class, with the following format:
class class_name { EG: Class student
access_specifier_1: {
member 1; charname;
access_specifier_2: int marks:
member2; float average;
… }s;
}object_names;
In the above eg: “S” is the object so we can access entire data of the student class by using this object. We discuss about the access specifiers in next concepts in detail.
Encapsulation: Wrapping up of a data in to single logical unit (i.e class) is called encapsulation. So writing class is known as encapsulation.
Polymorphism: Simply it one thing different actions let me explain consider one person (assume that person is one thing)he will exibit different actions depending on situations like his son called him daddy, and his father called him son, and his wife called him husband. I will explain how this concept is implemented in our C++ concepts in the upcoming chapters.
Inheritance: A key feature of C++ classes is inheritance. Inheritance allows creating classes which are derived from other classes, so that they automatically include some of its “parent’s” members, plus its own. We will the implementation of this concept in detail in the upcoming chapters.
Comments: comments are parts of the source code disregarded by the compiler. They simply do nothing. Their purpose is only to allow the programmer to insert notes or descriptions embedded within the source code.
C++ supports two ways to insert comments:
//line comment
/*block comment*/
The first of them, known as line comment, discards everything from where the pair of slash signs (//) is found up to the end of that same line. The second one, known as block comment, discards everything between the /* characters and the first appearance of the */ characters, with the possibility of including more than one line.
We are going to add comments to our second program:
If you include comments within the source code of your programs with out using the comment characters combinations //,/* or */, the compiler will take them as if they were C++ expressions, most likely causing one or several error messages when you compile it.
Preprocessor directives:
Preprocessor directives are lines included in the code of our programs that are not program statements but directives for the processor. These lines are always preceded by a pound sign (#). The processor executed before the actual compilation of code is generated by the statements.
These processor directives extend only across a single line of code. As soon as a new line character is found, the processor directive is considered to end. No semicolon (;) is expected at the end of a preprocessor directive. The only way a preprocessor directive can extend through more than one line is by preceding the new line character at the end of the line by a backslash (/)
Macro definitions (#define, #undef)
To define preprocessor macros we can use #define. Its formate is:
#define identifier replacement.
When the preprossor encounters this direccive , it replaces any occurrence of identifier in the rest of the code by replacement can be an expresson , a statement , a block or simply anything. The processor does not understand C++ , it simply replaces any occurrence of identifier by replacement.
#define TABLE_SIZE 100
int table 1 [TABLE_SIZE];
int table 2 [TABLE_SIZE];
After the preprocessor has replased TABLE_SIZE, the code becomes equivalent to:
Int table 1[100];
Int table 2 [100];
This use of #define as constant definer is already known by us from previous tutorials, but #define can work also with parameters to define function macros:
#define getmax(a,b) a>b?a:b
This would replace any occurrence of getmax followed by two arguments by the replacement expression, but also replasing each argument by its identifier, exactly as you would expect if it was a function:
//function macro
#include
Using namespace std;
#define getmax(a,b) ((a)>(b)?(a)b))
Int main()
{
Int x=5,y;
Y=getmax (x,2);
Cout << y << endl;
Cout<< getmax(7,x)<
} 5
6
Defined macros are not affected by block structure. A macro lasts until it is undefined with the #undef preprosseor directive:
#define table_size 100
Int table 1 [TABLE_SIZE];
#undef TABLE_SIZE
#define TABLE_SIZE 200
Int table 2[TABLE_SIZE];
This would generate the same code as:
Int table 1 [100];
Int table 2 [200];
Function macro definitions accept two special operators (# and ##) in the replacement sequence:
If the operator # is used before a parameter is used in the replacement sequence, that parameter is replaced by a string literal (as if it were enclosed between double quotes)
#define str (x) #x
Cout << str(test);
This would be translated into:
Cout << “test”;
The operator ## concatenates two arguments leaving no blank spaces between them:
#define glue(a,b) a## b
Glue(c,out) << ”test’’;
This would also be translated into:
Cout <<”test”;
Because preprocessor replacements happen before any C++ syntax check, macro definitions can be a tricky feature, but be careful: code that relies heavily on complicated macros may result obscure to other programmers, since the syntax they expect in C++.
Conditional inclusions (#ifdef, #ifdef, #if, #endif, #else and #elif)
These directives allow to include or discard part of the code of a program if a certain condition is met.
#ifdef allowes a section of a program to be compiled only if the macro that is specified as the parameter has been defined, no matter which its value is. For example:
#ifdef TABLE_SIZE
int table [TABLE_SIZE];
#endif
In this case , the line of code int table [TABLE_SIZE]; is only compiled if TABLE_SIZE was previously defined with #define, independently of its value. If it was not defined, that line will not be included in the program compilation.
#ifndef serves for the exact opposite: the code between #indef and #endif directives is only compiled if the specified identifier has not been previously defined. For example:
#ifndef TABLE_SIZE
#define TABLE_SIZE 100
#endif
Int table [TABLE_SIZE];
/* My second program in C++
With more comments*/
#include
Using namespace std;
Int main ()
{
Cout <<”Hello World!”; // prints Hello World!
Count <<”I’m a C++program”; //prints I’m a C++ program
return 0;
} Hellow World! I’m a C++ program
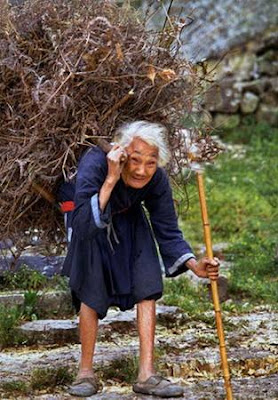.jpg)